Class, Objects, Constructor, Methods in Python :
What is Object-Oriented Programming (OOPs)?
In Python, Object-Oriented Programming (OOPs) is a way to write code that’s similar to how we deal with stuff in the real world. Instead of keeping data and actions apart, OOPs put them together by using concepts like Objects, Classes and Methods. It’s like keeping your ingredients and the recipe together, so you don’t accidentally mix things up.
To learn OOPs completely, we need to understand the following concepts :
- Class, Objects and Methods
- Polymorphism
- Encapsulation
- Inheritance
- Data Abstraction
In this particular OOPs series, we will look at Classes, Objects and Methods in detail.
What topics do we learn in this blog?
- Class
- Object
- Methods
- __init__constructor
- self parameter
Class, Objects, Constructor, Methods in Python
Let the Celebration Begin: A Magical Party with Sweets, Chocolates, and Return Gifts.
We have a party which is being organized by Nobita and the attendees of the party are Pokemon, ShinChan and Doraemon. At this party, as Pokemon is very fond of sweets he gets sweets, as ShinChan loves chocolates he gets chocolates and as Doraemon is a special friend of Nobita, he gets a return gift. To satisfy all these requirements we construct classes, objects and methods.
The Party Class – Main Code
In Python, a class serves as the magical blueprint. Here, we will create a party class to encapsulate the structure and behaviour of the celebration. In the party class, we can have different properties :
- names of people in the party,
- the role of that name whether they are a party attendee or a party organizer.
The class can also have different methods :
- __init__: To initialize the party attendees and to set their initial values.
- distribute_sweets: to distribute sweets to the party attendees.
- distribute_chocolates: to share chocolates with the party attendees.
- give_return_gifts: present return gifts only to special friends.
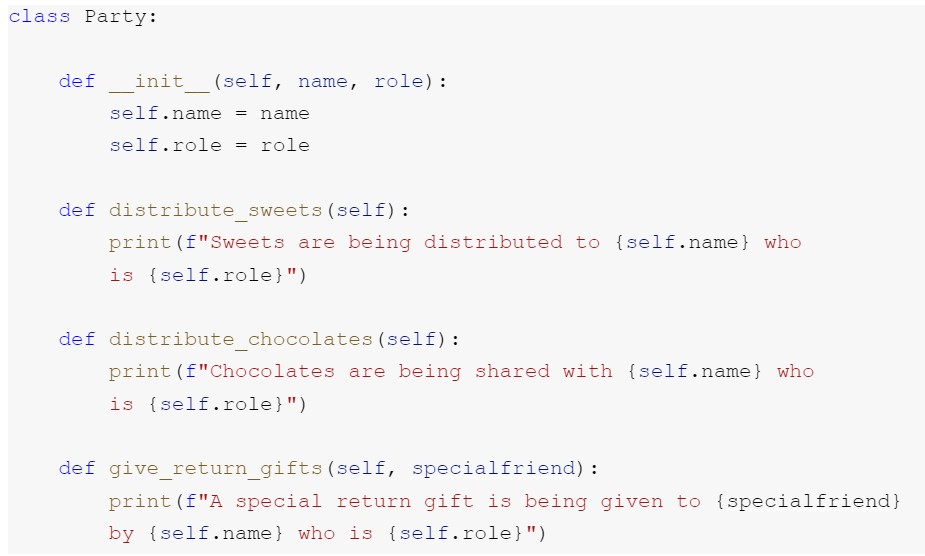
The Magic of Objects: Personalizing the Party Attendees
Objects bring life to our celebration. Here our party attendees serve as objects 🙂
- Pokemon – will attend the party and get the sweets
- Shinchan – will attend the party and get the chocolates
- Nobita – is throwing a party but wants to give a return gift to a special friend Doraemon
First, we create different objects in the Party class so later we can call these objects with different methods to carry out particular tasks.
Creating the objects
In our class we have two properties: name and role, so those two properties should be passed to all our objects.

Now let’s explore the wonders of the __init__ function.
The Enchanting init Function
In our main code, we have defined a special function __init__ within the Party class. There are three parameters in our function known as :
- self (representing the object being created)
- name (representing the name of the object)
- role (representing the role of the object)
The self.name and self.role properties assign the provided name and role to the object, establishing a personalized touch for the celebration.
Now let’s witness the magic of different methods in our class :
Calling Methods of the class with our objects so they can carry out different tasks :

Here if we observe, Nobita is giving a return gift to Doraemon, which is being passed as a parameter to the function: give_return_gifts(“Doraemon”)
The output of code after calling the methods with the objects

After calling the methods on our party attendees to perform actions. We see sweets being shared with Pokemon, chocolates being distributed to Shinchan, and a special return gift being given to Doraemon by Nobita.
Here, we have explored the concepts of classes, objects, methods, and the mystical __init__ function through the magic of a celebration. Now let’s see their definitions.
Class, Objects, Methods, init constructor definitions in Python
Class & Objects
In Python, a class is a blueprint or a template that defines the structure and behaviour of user-defined data. The class serves as a blueprint for creating multiple instances/objects that share similar characteristics and functionalities.
Methods
Methods are functions defined within a class that perform specific actions or behaviours associated with the objects of that class. They define how objects interact with each other and manipulate their data. Methods can access and modify the attributes (variables) of the class and provide functionality to the objects by adding more parameters inside it.
__init__ Constructor with Arguments and Parameters
The __init__ function, also known as the constructor, is a special pre-defined method inside Python that will be automatically invoked when an object is created from that class. It initializes the object’s attributes and sets their initial values. It allows us to define the state of an object when it is first created.
self parameter
The __init__ function and the other methods take the self parameter as their first argument. It represents the instance of the class itself and allows us to access and modify its attributes.
self need not always be named as self, we can also change their names but there should always be a parameter inside a method in the first position, as it represents the objects being called. Apart from self, we can also add other parameters to the methods to enhance the functionality of that method.
Reference link of the code
class,objects_methods_blog.ipynb
Summary
In this blog, Easy Explanation of OOPs Concepts in Python – Part 1: we have covered classes, objects, and methods in the OOPs. In the next blog, we will see different types of variables and methods in Python OOPs.
If you’re interested in learning more about Python, come join our organization!